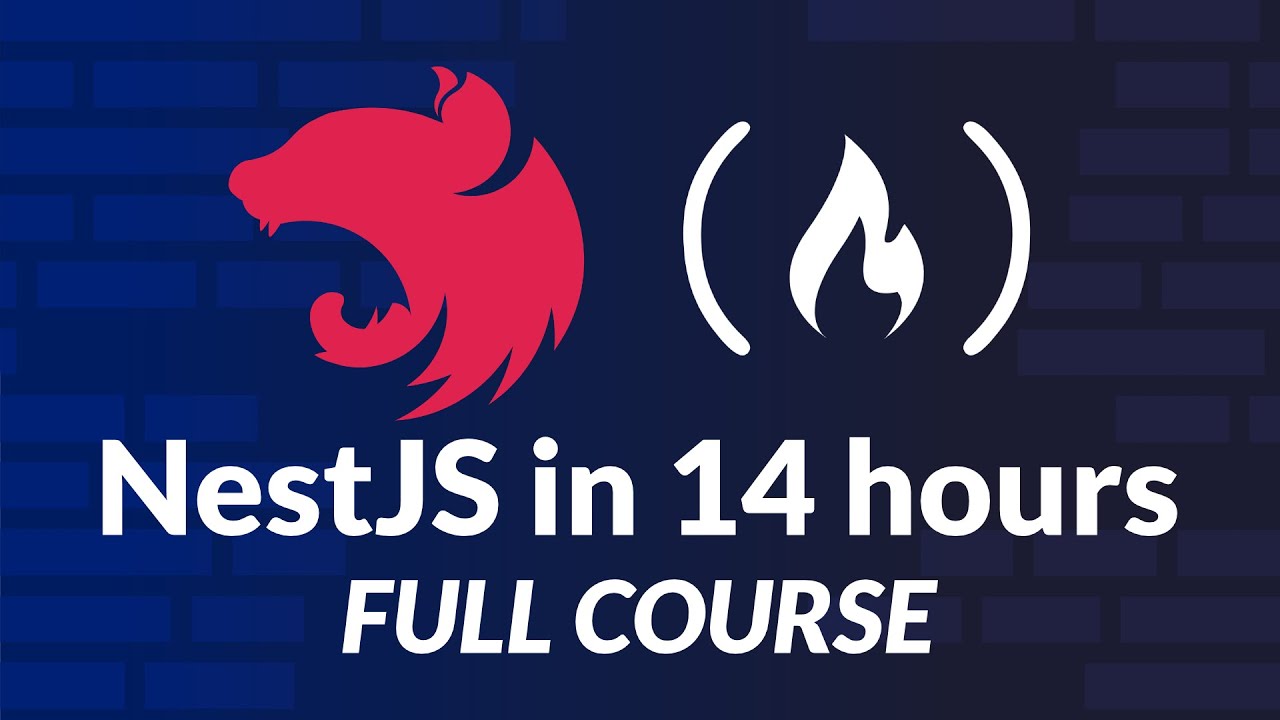
Learn NestJS – Complete Course
3/26/2024
Description
Learn NestJS in this comprehensive course for beginners. NestJS is a framework for building efficient, scalable Node.js web applications.
Code: https://github.com/HaiderMalik12/nestjs-fundamentals
Course resources e-book: https://www.haidermalik.dev/nestjs
Testing Starter Kit for Module 12: https://drive.google.com/file/d/1dU4ro10jZaIYAu32MwQFr4UHlY6GFu6O/view?usp=sharing
✏️ This course was developed by @haidermalik3402 . Check out more of his courses: https://www.udemy.com/user/5512f7602d2ad/
⭐️ Contents ⭐️
Module 0
Module 1
Module 2
Module 3
Module 4
Module 5
Module 6
Module 7
Module 8
Module 9
Module 10
Module 11
Module 12
Module 13
Module 14
Module 15
Module 16
Module 17
Module 18
Module 19
Module 20
Chapters
What is NestJS
3:01
Create NestJS Project
2:29
NestJS Directory Structure
1:30
Creating Controller
4:07
Creating a Service
7:02
Creating Module
6:18
Middleware
8:12
Exception Filter
10:28
Transform param using ParseIntPipe
5:03
Validate Request Body using class validator
3:59
Custom Providers
23:17
Injection Scopes
5:33
One To Many Relation
14:06
Establish Database Connection
8:37
Create an Entity
7:01
Create and Fetch records from Database
18:11
Pagination
8:50
One to One
6:30
Many to Many relation
19:37
User Signup
16:14
User Login
8:07
Authenticate User with Passport JWT
16:30
Role Based Authentication
22:09
Two Factor Authentication
30:50
API Key Authentication
15:11
Debug NestJS Application
4:08
Migrations
12:51
Seeding
12:11
Custom Configuration
22:27
Validate Env Variables
11:19
Hot Module Reloading
10:03
Swagger Setup
6:39
Document Signup Route
5:58
Create Schema using ApiProperty
4:26
Test JWT Authentication
8:46
Install MongoDB using Docker Compose
6:36
Connect with MongoDB
3:08
Create Schema
3:32
Save Record in Mongo Collection
8:12
Find and Delete
5:39
Populate
13:31
Configure Dev and Production Env
9:04
Push Source Code to Github Repo
5:16
Deploy NestJS Project to Railway
9:06
Install Dotenv to work with TypeORM migrations
4:36
Fixing Env Bugs
9:25
Getting started with Jest
7:37
Auto Mocking
17:51
SpyOn Function
10:36
Unit Test Controller
13:46
Unit Test Service
8:44
E2E Testing
13:39
Speedy Web Compiler with NestJS v10
8:33
Creating Websocket Server
8:34
Send Message from Frontend app
6:43
GraphQL Server Setup
7:55
Define Queries and Mutations
6:28
Resolve Queries
5:31
Resolve Mutations
4:53
Error Handling
3:39
Define Schema for Authentication
7:59
Resolve Auth Queries and Mutations
10:34
Apply Authentication using Auth Guard
19:19
Implement Real time Subscription
8:33
Unit Test Resolver
11:23
End to End Tesing GraphQL APIs
14:53
Server Side Caching using Apollo
12:01
Optimize Query Performance using Data Loader
15:10
Fetching Data from External REST API
6:50
Setup Prisma
3:29
Models and Migrations
3:43
Generate Prisma Client
2:35
Create, Find and FindOne
10:14
Update and Delete Operation
8:20
One to Many Relation
11:37
One to One Relation
6:39
Many to Many Relation
13:02
Bulk or Batch Operations
3:54
Implement Transaction using Nested Queries
8:27
Interactive Transactions
13:12
File Upload
10:04
Custom Decorator
5:51
Scheduling CRON Task with Nest.js
12:38
Cookies
8:22
Queues
12:40
Event Emitter
11:30
Streaming
5:42
Session
3:35