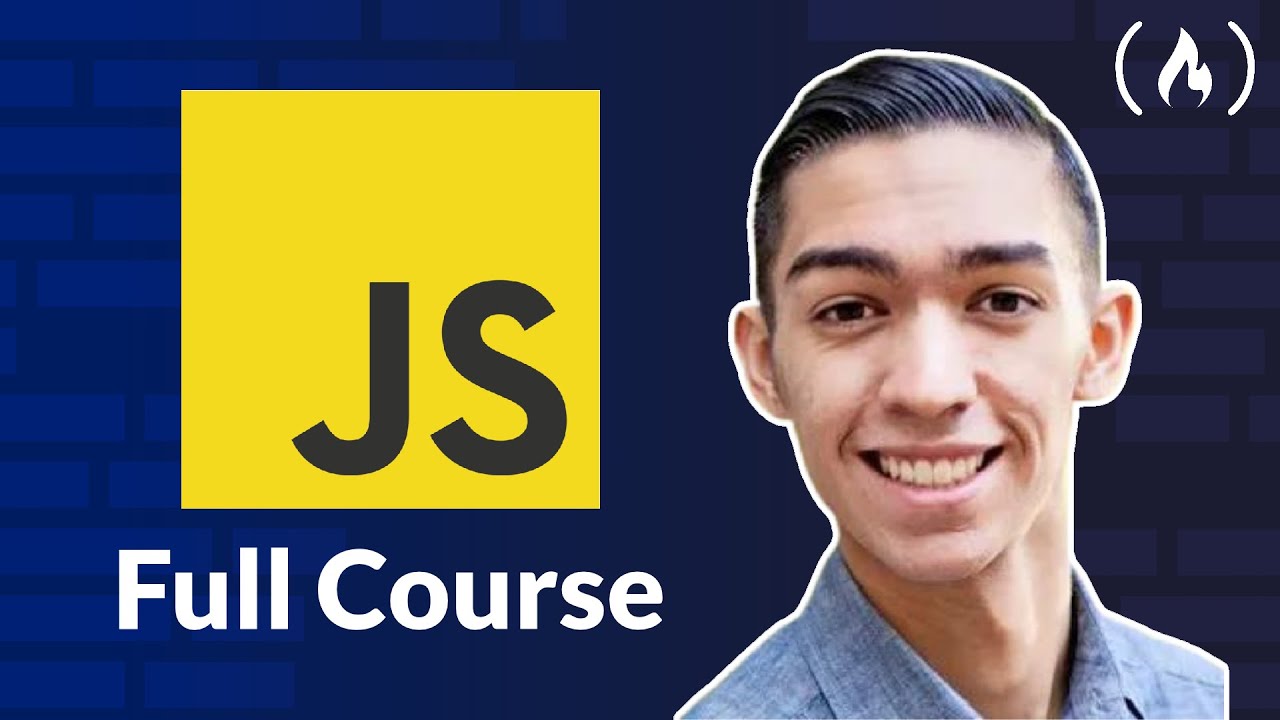
JavaScript Course for Beginners 2024
Description
Learn how to use the JavaScript programming language. This beginner's tutorial will teach you all the basics of JavaScript and also includes quiz sections.
Code for course: https://github.com/stevenGarciaDev/javascript-for-beginners-notes
Course developed by @StevenCodeCraft
Steven's LinkedIn: https://www.linkedin.com/in/stevengarciadev/
⭐️ Contents ⭐️
Section 1: Getting Started
Section 2: JavaScript Variables
Section 3: JavaScript Operators
Section 4: Control Flow
Section 5: JavaScript Objects
Section 6: JavaScript Arrays
Section 7: JavaScript Functions
🎉 Thanks to our Champion and Sponsor supporters:
👾 davthecoder
👾 jedi-or-sith
👾 南宮千影
👾 Agustín Kussrow
👾 Nattira Maneerat
👾 Heather Wcislo
👾 Serhiy Kalinets
👾 Justin Hual
👾 Otis Morgan
👾 Oscar Rahnama
--
Learn to code for free and get a developer job: https://www.freecodecamp.org
Read hundreds of articles on programming: https://freecodecamp.org/news